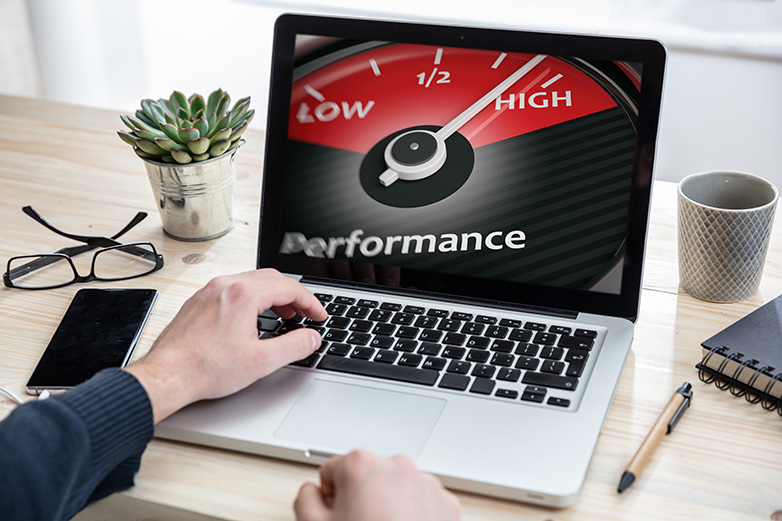
Optimizing Flutter App Performance: A Comprehensive Guide
Flutter, Google’s UI toolkit, has gained immense popularity due to its ability to create high-performance cross-platform applications. However, even with Flutter’s advantages, performance optimization is crucial to deliver a seamless user experience.
Here are some key strategies to optimize your Flutter app’s performance:
1. Minimize Widget Rebuilds
- Use
const
constructors: Declare widgets asconst
whenever possible to prevent unnecessary rebuilds. - Leverage
ListView.builder
: UseListView.builder
for large lists to improve scrolling performance. - Utilize
AutomaticKeepAliveClientMixin
: Keep widgets alive in the background to avoid unnecessary rebuilds.
2. Optimize Images
- Compress images: Use tools like ImageOptim or TinyPNG to reduce image file sizes.
- Use
Image.asset
withcacheWidth
andcacheHeight
: Pre-cache images to improve loading times. - Consider using
CachedNetworkImage
: For network-based images, useCachedNetworkImage
to cache and reuse images.
3. Reduce Layout Complexity
- Minimize nested widgets: Avoid excessive nesting of widgets, as it can impact layout performance.
- Use
LayoutBuilder
for dynamic layouts: UseLayoutBuilder
to create dynamic layouts based on available space. - Profile your app: Use Flutter’s profiling tools to identify performance bottlenecks.
4. Optimize State Management
- Choose the right state management solution: Consider options like Provider, BLoC, or GetX based on your project’s complexity.
- Avoid unnecessary state updates: Only update state when necessary to prevent unnecessary rebuilds.
- Use
useMemoized
oruseSelector
for derived data: Memoize derived data to prevent redundant calculations.
5. Leverage Flutter’s Performance Tools
- Use the Flutter DevTools: Analyze your app’s performance metrics, memory usage, and CPU usage.
- Profile your app in release mode: Ensure your app is optimized for production environments.
6. Consider Asynchronous Operations
- Use
Future
andasync/await
: Perform long-running tasks asynchronously to prevent UI freezes. - Utilize
isolate
for CPU-intensive tasks: Offload CPU-intensive tasks to separate isolates to avoid blocking the main thread.
By following these guidelines, you can significantly improve the performance of your Flutter app, providing a smoother and more enjoyable user experience.
Comments