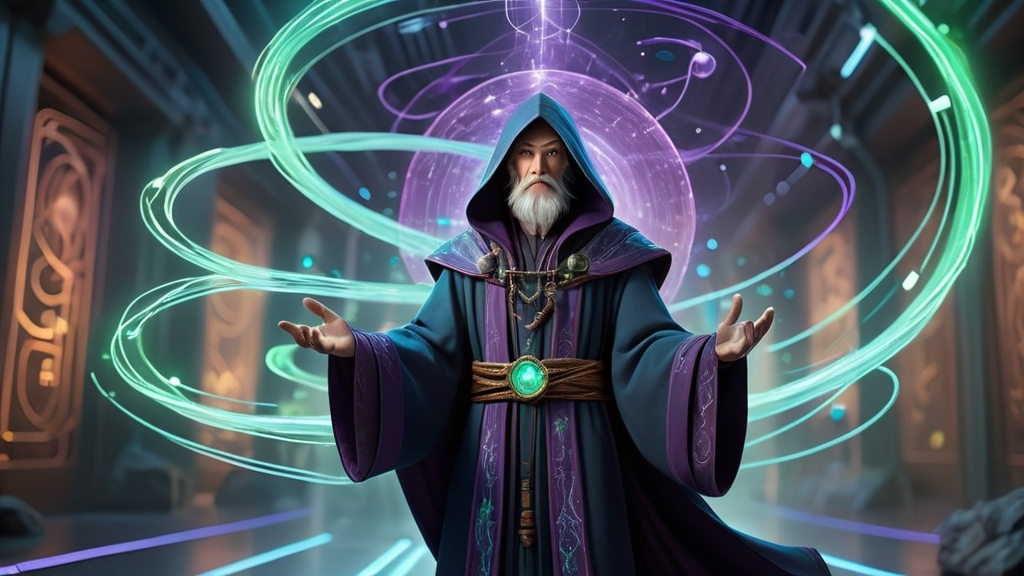
๐งโโ๏ธ Flutter's Server-Side Sorcery: Mastering Server-Loaded Widgets
Unleash the Power of Dynamic, Data-Driven UIs
Welcome, Flutter magicians! Today, weโre diving into the mystical realm of server-loaded widgets โ where your appโs UI becomes a canvas painted by data from the cloud. โ๏ธโจ Get ready to create Flutter apps that adapt and transform based on server-side configurations!
๐ฎ The Magic of Server-Loaded Widgets
Server-loaded widgets allow you to:
- ๐ Update your UI without app store releases
- ๐จ A/B test different layouts effortlessly
- ๐ Create dynamic, content-driven experiences
- ๐ ๏ธ Customize UI based on user data or preferences
Letโs unlock the secrets to this powerful technique!
๐งช The Ingredients
Before we start casting spells, letโs gather our magical components:
- ๐ก API endpoint serving widget configurations
- ๐๏ธ JSON structure defining widget layouts
- ๐ Widget interpreter in Flutter
- ๐๏ธ Dynamic widget builder
๐ช The Incantation: Step-by-Step Guide
1. ๐ Define Your Widget JSON Structure
Create a consistent JSON structure to define your widgets:
{
"type": "container",
"properties": {
"color": "#FFFF00"
},
"children": [
{
"type": "text",
"properties": {
"text": "Hello, Server-loaded World!",
"style": {
"fontSize": 20,
"color": "#000000"
}
}
}
]
}
2. ๐ Fetch Widget Configurations
Use http
package to fetch widget configurations:
import 'package:http/http.dart' as http;
import 'dart:convert';
Future<Map<String, dynamic>> fetchWidgetConfig() async {
final response = await http.get(Uri.parse('https://api.example.com/widget-config'));
if (response.statusCode == 200) {
return json.decode(response.body);
} else {
throw Exception('Failed to load widget configuration');
}
}
3. ๐ฎ Create a Widget Interpreter
Develop a function to interpret JSON and build widgets:
import 'package:flutter/material.dart';
Widget buildWidgetFromJson(Map<String, dynamic> json) {
switch (json['type']) {
case 'container':
return Container(
color: Color(int.parse(json['properties']['color'].substring(1, 7), radix: 16) + 0xFF000000),
child: buildWidgetFromJson(json['children'][0]),
);
case 'text':
return Text(
json['properties']['text'],
style: TextStyle(
fontSize: json['properties']['style']['fontSize'].toDouble(),
color: Color(int.parse(json['properties']['style']['color'].substring(1, 7), radix: 16) + 0xFF000000),
),
);
// Add more cases for other widget types
default:
return SizedBox.shrink();
}
}
4. ๐๏ธ Implement a Dynamic Widget Builder
Create a widget that fetches and builds the server-loaded widget:
class ServerLoadedWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
return FutureBuilder<Map<String, dynamic>>(
future: fetchWidgetConfig(),
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.done) {
if (snapshot.hasData) {
return buildWidgetFromJson(snapshot.data!);
} else if (snapshot.hasError) {
return Text('Error: ${snapshot.error}');
}
}
return CircularProgressIndicator();
},
);
}
}
5. ๐ Use Your Server-Loaded Widget
Integrate the dynamic widget into your app:
class MyHomePage extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Server-Loaded Widget Demo')),
body: Center(
child: ServerLoadedWidget(),
),
);
}
}
๐ง Advanced Techniques
1. ๐ Caching and Offline Support
Implement caching to improve performance and enable offline use:
import 'package:shared_preferences/shared_preferences.dart';
Future<Map<String, dynamic>> fetchWidgetConfig() async {
SharedPreferences prefs = await SharedPreferences.getInstance();
try {
final response = await http.get(Uri.parse('https://api.example.com/widget-config'));
if (response.statusCode == 200) {
final widgetConfig = json.decode(response.body);
prefs.setString('widget_config', json.encode(widgetConfig));
return widgetConfig;
}
} catch (e) {
print('Error fetching config: $e');
}
// Return cached config if fetch fails
final cachedConfig = prefs.getString('widget_config');
if (cachedConfig != null) {
return json.decode(cachedConfig);
}
throw Exception('Failed to load widget configuration');
}
2. ๐ Hot Reloading Server Widgets
Implement a mechanism to check for updates and hot reload:
class HotReloadableServerWidget extends StatefulWidget {
@override
_HotReloadableServerWidgetState createState() => _HotReloadableServerWidgetState();
}
class _HotReloadableServerWidgetState extends State<HotReloadableServerWidget> {
Map<String, dynamic>? _widgetConfig;
@override
void initState() {
super.initState();
_loadWidgetConfig();
// Check for updates every 5 minutes
Timer.periodic(Duration(minutes: 5), (_) => _loadWidgetConfig());
}
Future<void> _loadWidgetConfig() async {
try {
final newConfig = await fetchWidgetConfig();
if (!mapEquals(_widgetConfig, newConfig)) {
setState(() {
_widgetConfig = newConfig;
});
}
} catch (e) {
print('Error reloading widget config: $e');
}
}
@override
Widget build(BuildContext context) {
return _widgetConfig == null
? CircularProgressIndicator()
: buildWidgetFromJson(_widgetConfig!);
}
}
3. ๐ญ A/B Testing with Server-Loaded Widgets
Implement A/B testing by serving different configurations:
Future<Map<String, dynamic>> fetchABTestWidgetConfig() async {
final userId = await getUserId(); // Implement this method
final response = await http.get(
Uri.parse('https://api.example.com/ab-test-widget-config'),
headers: {'User-ID': userId},
);
if (response.statusCode == 200) {
return json.decode(response.body);
} else {
throw Exception('Failed to load A/B test configuration');
}
}
๐งโโ๏ธ Best Practices for Server-Loaded Widgets
- ๐ก๏ธ Implement error handling and fallback widgets
- ๐ฆ Use versioning in your widget configurations
- ๐๏ธโโ๏ธ Optimize payload size to reduce load times
- ๐ Monitor usage and gather analytics
- ๐ Ensure security by validating server responses
๐ Conclusion: The Future is Server-Loaded!
Server-loaded widgets open up a world of possibilities for creating dynamic, adaptable Flutter apps. By mastering this technique, youโre not just building apps โ youโre creating living, breathing digital experiences that can evolve without the need for constant app updates.
Remember, with great power comes great responsibility. Use server-loaded widgets wisely, always keeping user experience and performance in mind.
Now go forth and weave some server-side magic into your Flutter apps! May your widgets be ever dynamic and your users ever delighted! ๐โจ
Comments