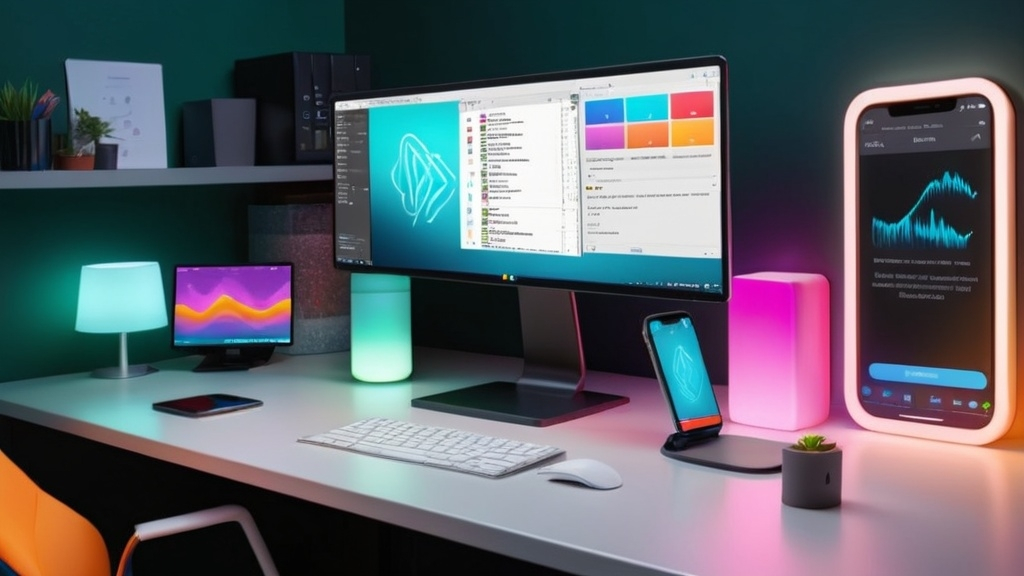
π¨ Flutter Layout Mastery: Crafting Pixel-Perfect UIs
Unlock the Secrets to Clean, Responsive Layouts
Are you tired of battling oversized widgets, misaligned elements, and the dreaded yellow-black overflow warnings? Fear not, fellow Flutter enthusiast! This guide will equip you with the principles and tricks to create harmonious, pixel-perfect layouts that adapt to any screen size. Letβs dive in and master the art of Flutter layouts! π
π§± Core Layout Principles
1. Embrace Constraint-Based Layouts
Flutterβs layout system is built on constraints. Understanding this is key to mastering layouts:
- Parents give constraints to children (min/max width and height)
- Children determine their size within these constraints
- Parents then position their children
ConstrainedBox(
constraints: BoxConstraints(maxWidth: 200, minHeight: 100),
child: MyWidget(),
)
2. Use Flex for Flexibility
Flex-based widgets (Row
, Column
) are your best friends for creating adaptive layouts:
Expanded
andFlexible
widgets help distribute space- Use
flex
parameter to control space distribution
Row(
children: [
Expanded(flex: 2, child: BlueBox()),
Expanded(child: RedBox()),
],
)
3. Leverage Intrinsic Sizing
When you need a parent to size based on its children:
IntrinsicHeight
andIntrinsicWidth
can be lifesavers- Use sparingly, as they can be computationally expensive
IntrinsicHeight(
child: Row(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [
VariableHeightWidget(),
FixedSizeWidget(),
],
),
)
π© Tricks for Clean Layouts
1. π The Invisible Hero: SizedBox
Use SizedBox
for precise spacing and as an invisible placeholder:
Column(
children: [
Text('Hello'),
SizedBox(height: 16), // Consistent vertical spacing
Text('World'),
],
)
2. π Overflow Control with SingleChildScrollView
Wrap your content in a SingleChildScrollView
to handle potential overflow:
SingleChildScrollView(
child: Column(
children: [
// Lots of widgets that might overflow
],
),
)
3. π AspectRatio for Consistent Proportions
Maintain widget proportions across different screen sizes:
AspectRatio(
aspectRatio: 16 / 9,
child: MyVideoPlayer(),
)
4. πΌ LayoutBuilder for Context-Aware Widgets
Adapt your layout based on the available space:
LayoutBuilder(
builder: (context, constraints) {
if (constraints.maxWidth > 600) {
return WideLayout();
} else {
return NarrowLayout();
}
},
)
5. ποΈ FractionallySizedBox for Responsive Sizing
Size widgets as a fraction of their parent:
FractionallySizedBox(
widthFactor: 0.8, // 80% of parent width
child: Container(color: Colors.blue),
)
π« Common Pitfalls and How to Avoid Them
1. Infinite Height in ScrollView
Problem: Using ListView
or Column
inside a ScrollView
can lead to infinite height issues.
Solution: Use shrinkWrap: true
for nested scrollable, or better, restructure your layout.
ListView(
shrinkWrap: true,
children: [/*...*/],
)
2. Overflowing Flex
Problem: Children of Row
or Column
overflow the parent.
Solution: Wrap children with Expanded
or use Flexible
with fit: FlexFit.loose
.
Row(
children: [
Flexible(
fit: FlexFit.loose,
child: MyPotentiallyWideWidget(),
),
],
)
3. Text Overflow
Problem: Text overflows its container.
Solution: Use overflow
property or wrap in Flexible
widget.
Flexible(
child: Text(
'Very long text...',
overflow: TextOverflow.ellipsis,
),
)
π Putting It All Together
Remember, creating clean layouts is an art form. Hereβs a checklist for layout success:
- β Start with proper constraints
- β Use flex-based layouts for adaptivity
- β Control overflow with scrolling when necessary
- β
Leverage
SizedBox
for precise spacing - β
Use
LayoutBuilder
for context-aware designs - β Test on various screen sizes
By applying these principles and tricks, youβll be crafting pixel-perfect, responsive layouts in no time. Happy Flutter-ing! ππ¨βπ»π©βπ»
Comments