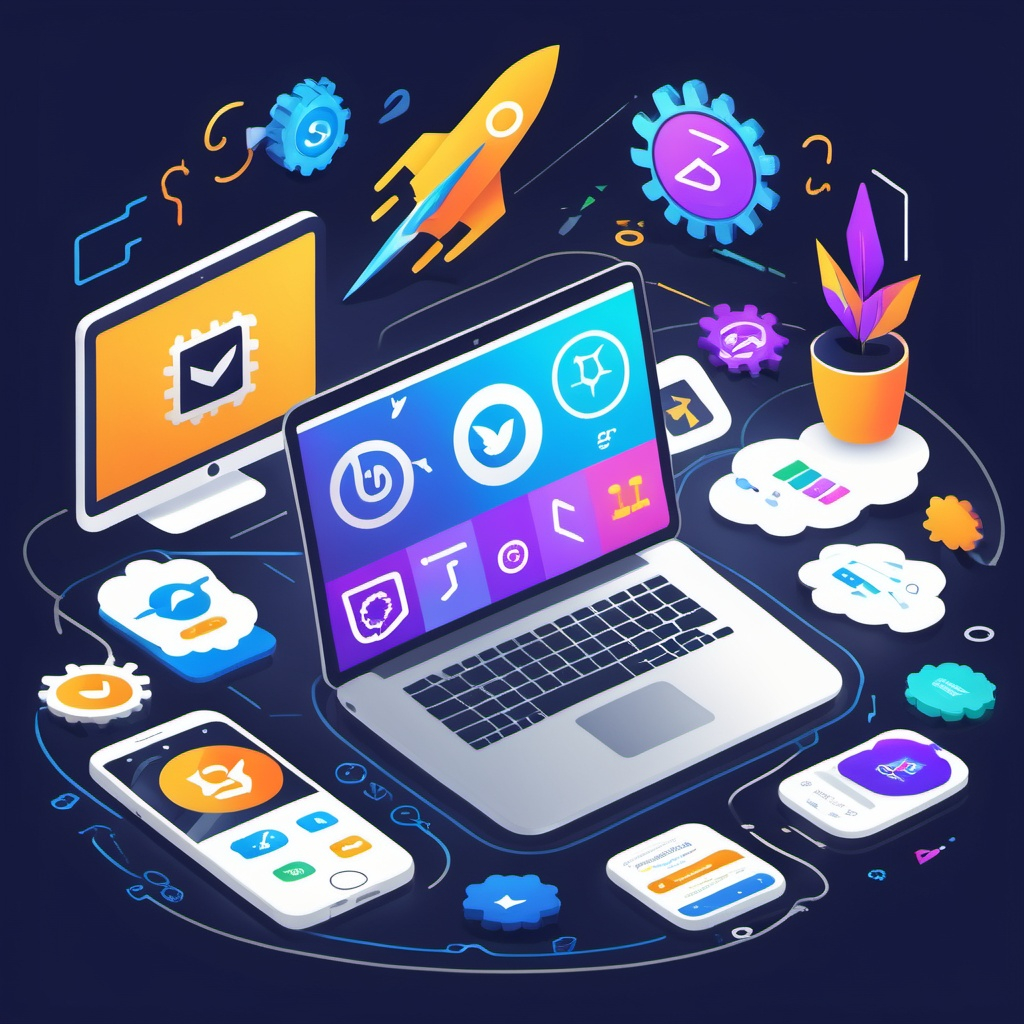
10 Flutter Tricks to Supercharge Your Development
Flutter has taken the mobile development world by storm, offering a powerful framework for building beautiful, natively compiled applications for mobile, web, and desktop from a single codebase. Whether you’re a Flutter newbie or a seasoned pro, these 10 tricks will help you write cleaner, more efficient code and boost your productivity. Let’s dive in!
1. Use const Constructors
Whenever possible, use const
constructors. This can significantly improve your app’s performance by allowing Flutter to optimize memory usage.
// Instead of this
final myWidget = MyWidget();
// Do this
const myWidget = MyWidget();
This simple change tells Flutter that this widget is immutable and can be reused across builds.
2. Leverage the Cascade Notation
The cascade notation (..
) allows you to perform multiple operations on the same object without repeating the object name.
final paint = Paint()
..color = Colors.black
..strokeCap = StrokeCap.round
..strokeWidth = 5.0;
This is much cleaner than assigning each property separately.
3. Use Named Parameters for Clarity
When creating functions or constructors with multiple parameters, use named parameters to make your code more readable and less error-prone.
void createUser({
required String name,
required String email,
int age = 0,
}) {
// Function body
}
// Usage
createUser(name: 'John Doe', email: '[email protected]', age: 30);
This makes it clear what each argument represents and allows for optional parameters with default values.
4. Implement the BuildContext
Extension
Create extensions on BuildContext
to access commonly used properties or methods more easily.
extension BuildContextExtensions on BuildContext {
ThemeData get theme => Theme.of(this);
TextTheme get textTheme => theme.textTheme;
ColorScheme get colorScheme => theme.colorScheme;
Size get screenSize => MediaQuery.of(this).size;
// Add more as needed
}
// Usage
Text(
'Hello',
style: context.textTheme.headline6,
color: context.colorScheme.primary,
);
This makes your code cleaner and more concise.
5. Use if
in Collection Literals
Flutter allows you to use if
statements directly within collection literals, which is great for conditional UI elements.
return Column(
children: [
Text('Always visible'),
if (isLoggedIn) Text('Welcome back!'),
...commonWidgets,
],
);
This is more readable than using ternary operators or separate variables.
6. Leverage AnimatedBuilder
for Performance
When animating parts of your UI, use AnimatedBuilder
to rebuild only the parts that change, rather than the entire widget tree.
AnimatedBuilder(
animation: _controller,
builder: (context, child) {
return Transform.rotate(
angle: _controller.value * 2.0 * pi,
child: child,
);
},
child: const MyComplexWidget(),
)
This ensures that MyComplexWidget
is not rebuilt on every animation frame.
7. Use const
for Widget Keys
When using keys, make them const
to improve performance:
const Key('my_unique_key')
This allows Flutter to optimize memory usage and widget rebuilds.
8. Implement Custom Lint Rules
Create custom lint rules to enforce coding standards specific to your project or team.
# In analysis_options.yaml
linter:
rules:
- always_specify_types
- avoid_print
# Add your custom rules here
This helps maintain code quality across your team.
9. Utilize the Visibility
Widget
Instead of using ternary operators to show/hide widgets, use the Visibility
widget:
Visibility(
visible: shouldShow,
child: MyWidget(),
)
This is more readable and allows you to specify a replacement widget when the main one is hidden.
10. Implement Lazy Loading with FutureBuilder
Use FutureBuilder
to implement lazy loading of data, improving your app’s initial load time:
FutureBuilder<List<Item>>(
future: fetchItems(),
builder: (context, snapshot) {
if (snapshot.hasData) {
return ListView(children: snapshot.data!.map((item) => ItemWidget(item)).toList());
} else if (snapshot.hasError) {
return Text('Error: ${snapshot.error}');
}
// By default, show a loading spinner.
return const CircularProgressIndicator();
},
)
This pattern is great for loading data asynchronously without blocking the UI.
Bonus Tip: Use the DevTools
While not a coding trick per se, mastering Flutter’s DevTools can significantly boost your productivity. It offers performance profiling, widget inspection, and much more.
By incorporating these tricks into your Flutter development workflow, you’ll write cleaner, more efficient code and build better apps. Remember, the key to mastering Flutter is practice and continuous learning. Keep exploring, and happy coding!
Stay tuned to Tech Bench: Code & Security for more Flutter tips and tricks!
Comments